Building a Weather App in React Native with Expo: A Step-by-Step Guide
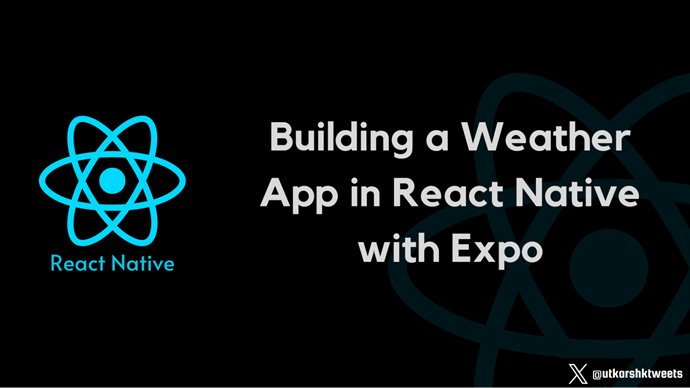
In this detailed guide, we will walk you through the process of building a weather app in React Native using Expo. React Native is a popular framework for developing cross-platform mobile applications, and Expo simplifies the development process.
By the end of this guide, you will have a fully functional weather app that can display weather information for different cities. If you want to learn basics of React Native, refer to our react native series
Prerequisites
Before we start, make sure you have the following prerequisites in place:
- Node.js and npm (Node Package Manager) installed on your machine.
- Expo CLI installed globally. You can install it using the following command:
npm install -g expo-cli
- A code editor, such as Visual Studio Code.
Step 1: Create a New Expo Project
Begin by creating a new Expo project. Open your terminal and run the following command:
expo init WeatherApp
Follow the on-screen instructions to configure your project. You can choose a template and set a project name. Once your project is created, navigate to the project directory:
cd WeatherApp
Step 2: Install Dependencies
Next, install the required dependencies for your project. You will need Axios to make API requests and React Navigation to handle navigation between screens.
Run the following commands to install these packages:
npm install axios react-navigation react-navigation-stack
Step 3: Create Screens
In your project directory, create a folder named `screens`, create two files inside of it:
`HomeScreen.js` and `SearchScreen.js`.
These screens will serve as the main components of your weather app.
`HomeScreen.js` (Home Screen)
Create the `HomeScreen.js` file with the following content:
import React from "react";
import { View, Text, Button, StyleSheet } from "react-native";
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center",
alignItems: "center",
backgroundColor: "#e3dbd5",
},
heading: {
fontSize: 24,
fontWeight: "bold",
marginBottom: 16,
color: "black",
},
});
const HomeScreen = ({ navigation }) => {
return (
<View style={styles.container}>
<Text style={styles.heading}>Welcome to Weather App</Text>
<Button
title="Search City"
onPress={() => navigation.navigate("Search")}
/>
</View>
);
};
export default HomeScreen;
User Interface Setup:
The "HomeScreen" component is designed for a weather app and sets up the initial user interface. It includes a centered view with a welcome message and a button that allows users to navigate to the "Search" screen.
Navigation Integration:
The component utilizes the `navigation` prop to enable navigation between different screens. When users click the "Search City" button, it triggers navigation to the "Search" screen, enhancing the user experience.
Styling:
Styling is achieved using a `StyleSheet`, defining the appearance of the components, such as the background color, heading style, and button appearance, ensuring a visually pleasing and user-friendly design.
`SearchScreen.js` (Search Screen)
Create the `SearchScreen.js` file with the following content:
import React, { useState } from "react";
import { View, Text, TextInput, Button, StyleSheet } from "react-native";
import { fetchWeatherData } from "../api/weather";
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center",
alignItems: "center",
backgroundColor: "#e3dbd5",
},
input: {
marginBottom: 10,
paddingHorizontal: 10,
width: 200,
height: 40,
borderColor: "gray",
borderWidth: 1,
},
weatherText: {
fontSize: 20,
fontWeight: "bold",
},
});
const SearchScreen = ({ navigation }) => {
const [city, setCity] = useState("");
const [weatherData, setWeatherData] = useState(null);
const handleSearch = async () => {
try {
const data = await fetchWeatherData(city);
setWeatherData(data);
} catch (error) {
// Handle the error
}
};
return (
<View style={styles.container}>
<TextInput
style={styles.input}
placeholder="Enter a city"
onChangeText={(text) => setCity(text)}
value={city}
/>
<Button title="Search" onPress={handleSearch} />
{weatherData && (
<View>
<Text style={styles.weatherText}>
{weatherData.name}, {weatherData.sys.country}: {weatherData.main.temp}°C
</Text>
</View>
)}
</View>
);
};
export default SearchScreen;
User Input and API Interaction:
The "SearchScreen" component allows users to input a city name. When users click the "Search" button, it triggers an asynchronous function, handleSearch
, which communicates with an API to fetch weather data for the specified city.
Dynamic Rendering:
The component dynamically renders the user interface based on the retrieved weather data. If data is available, it displays information like city name, country, and temperature in Celsius, enhancing the app's functionality.
State Management:
The component manages the state using the useState
hook to handle user input (city name) and store fetched weather data, ensuring a responsive and interactive user experience.
Step 4: Create API Request Function
To access weather data through OpenWeather API, follow these simple steps:
Visit the OpenWeather website and create an account.
Once registered, log in to your account.
After logging in, go to the API keys section.
Generate a new API key by clicking the "Generate" button.
You'll receive a unique API key. Keep it secure and never share it publicly.
Now, you can use this API key in your applications to fetch weather data, like in our Weather App project, to access real-time weather information for different locations.
Now, Create a folder called `api` in your project directory.
Inside this folder, create a file named `weather.js`.
This file will contain the function to fetch weather data using an API.
`api/weather.js` (API Request Function)
Create the `weather.js` file with the following content:
export const fetchWeatherData = async (city) => {
try {
const apiKey = "YOUR_API_KEY";
const response = await fetch(`https://api.openweathermap.org/data/2.5/weather?q=${city}&units=metric&appid=${apiKey}`);
const data = await response.json();
return data;
} catch (error) {
throw error;
}
};
API Data Retrieval:
The fetchWeatherData
function is responsible for making an asynchronous API request to OpenWeatherMap. It retrieves weather-related data for a specified city by constructing the API URL with the city name and an API key.
API Key Requirement:
It requires an API key for authentication, which should be replaced with a valid key. The key is crucial for accessing the weather data and should be kept secure.
Error Handling:
The function includes error handling, which catches and rethrows errors that may occur during the API request. Proper error handling is essential to ensure that the application gracefully handles unexpected issues when fetching data.
Replace `'YOUR_API_KEY'` with your OpenWeatherMap API key.
Step 5: Set Up Navigation
In your `App.js` file, set up navigation using React Navigation.
You'll need to import the necessary components and create a navigation stack to switch between `HomeScreen` and `SearchScreen`.
`App.js`
import React from "react";
import { NavigationContainer } from "@react-navigation/native";
import { createStackNavigator } from "@react-navigation/stack";
import HomeScreen from "./screens/HomeScreen";
import SearchScreen from "./screens/SearchScreen";
const Stack = createStackNavigator();
export default function App() {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName="Home">
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Search" component={SearchScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
React Native Navigation Configuration:
This code sets up the primary entry point for a React Native app and configures navigation using the `@react-navigation` library. It defines a stack-based navigation structure for the app.
Screen Definitions:
The code defines two screens, "Home" and "Search," within the app's navigation structure. "Home" is set as the initial route, while "Search" is available for users to navigate to.
Navigation Container:
It wraps the entire application with the `NavigationContainer`, which provides a context for navigation. This structure enables seamless navigation between different screens within the app.
Step 6: Start Your App
Now, you're ready to run your weather app. Start your app using the following command:
expo start
A new window will open in your browser, and you can choose to run your app on an emulator, physical device, or a web browser.
Step 7: Test and Explore
You can now test your weather app by searching for cities and viewing the weather information on the `SearchScreen`.
You have successfully created a basic weather app in React Native with Expo!
Feel free to expand and enhance your app by adding more features, styling, and additional screens to make it a fully functional weather app.
Conclusion
Creating a weather app in React Native with Expo is just the beginning of your mobile app development journey. Building projects like this helps you strengthen your React Native skills and opens the door to creating more complex applications in the future. By exploring different APIs, adding features such as location-based weather, and refining your app's design, you can take your skills to the next level. Whether you're building weather apps, task managers, or e-commerce platforms, React Native empowers you to create powerful, cross-platform applications with ease. Congratulations on building your weather app, and we look forward to seeing the exciting projects you'll develop next.
Happy Coding!