Building Your First React Native App
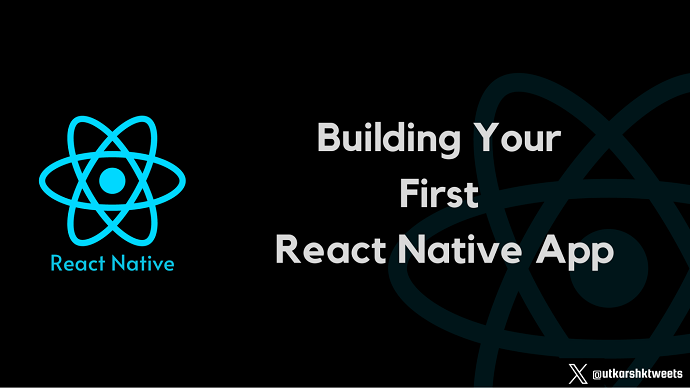
In the realm of mobile app development, React Native offers a powerful solution for crafting applications that seamlessly run on both iOS and Android platforms. When you embark on your journey to build a React Native app, you have two main avenues to choose from: the Standard Approach and Expo.
In this blog, we'll delve into both methods, explore the advantages of using Expo, and guide you through creating your very first React Native app with Expo.
The Standard Approach: Setting Up a React Native Project
Before we dive into the world of Expo, let's first acquaint ourselves with the traditional method of setting up a React Native project. While this approach provides more control and customization, it involves a few additional steps.
Step 1: Installing React Native Globally
Your journey begins with the installation of the React Native Command Line Interface (CLI), a global tool that empowers you to create, manage, and run React Native projects on your local machine.
Open your terminal and execute the following command to install it globally:
npm install -g react-native
This CLI tool is your trusty companion throughout your React Native adventure.
Step 2: Creating a React Native Project
Now, let's give birth to your React Native project. In your terminal, navigate to your chosen project directory and execute the following command:
react-native init MyReactNativeApp
This command initializes a fresh React Native project, aptly named "MyReactNativeApp."
It lays the foundation for your project by establishing the necessary structure and installing vital dependencies.
The Advantages of the Standard Approach
The Standard approach offers a range of advantages:
Full Control:
By starting your project from scratch, you wield complete control over its configuration. This empowers you to customize your project to precisely match your requirements.
Access to Native Modules:
In the standard approach, you have the freedom to add and access native modules crafted in languages like Swift, Objective-C, Java, or Kotlin.
Introducing Expo: Simplified React Native Development
Expo is a robust toolkit for React Native app development. It streamlines the development process, making it an excellent choice for newcomers and those who wish to eliminate the complexities of native module linking and manual configuration. With Expo, you can focus on the exciting journey of building amazing apps without grappling with intricate development nuances.
For more information, refer to Expo Documentation
The Advantages of Using Expo
Embracing Expo comes with several notable advantages:
Quick Setup:
Expo simplifies the creation of a new React Native project. You can sidestep the challenges of configuring native modules and manual setup, allowing you to dive into development swiftly.
Over-the-Air (OTA) Updates:
Expo grants you the power to update your app seamlessly over the air. This means you can deliver new features and squash bugs without requiring users to install updates via app stores.
Rich Set of Components:
Expo boasts a generous collection of pre-built components and libraries, making it easier to develop various features within your app.
Simplified Development:
Testing your app on physical devices becomes a breeze with Expo. By scanning a QR code, you can simplify the testing process. Additionally, you can run your app in the Expo client app, available on Android and iOS.
Getting Started with Expo
Now that we're well-versed in the merits of Expo, it's time to take our first steps into the world of React Native development with Expo.
Before we proceed, ensure you have Expo CLI installed globally on your system. To do so, open your terminal and execute the following command:
npm install -g expo-cli
This installation equips you with the Expo CLI, a potent tool for creating and managing Expo projects.
Creating Your First React Native Project with Expo
Now, let's utilize Expo to craft your very first React Native app. The process with Expo is seamless and intuitive, allowing you to start your project swiftly.
1. Open your terminal and navigate to the directory where you intend to create your project.
2. Execute the following command to initiate a new Expo project:
expo init MyExpoApp
The Expo CLI will guide you through the project setup. You'll be offered various project templates to choose from, including a blank template for a clean slate, a minimal template, and templates pre-configured with navigation features.
3. After creating the project, navigate to its directory using the `cd` command:
cd MyExpoApp
You are now situated within the root directory of your Expo project.
Understanding the Project Structure
Before we dive into coding, let's take a moment to explore the project structure. Gaining an understanding of how Expo organizes files and folders is vital for efficient development.
The Project Root
The root directory of your Expo project encompasses essential files and folders:
`node_modules/`: This directory houses all the dependencies required for your project, including the Expo SDK and pre-built components.
`App.js`: This file serves as the entry point for your application. It contains the root component of your app.
`package.json`: The `package.json` file lists the dependencies for your project and provides scripts for various development tasks.
App Components
Expo apps, similar to standard React Native apps, are constructed using components. Components are the fundamental building blocks of your app's user interface, and Expo's structure aligns with this concept. Components are organized within the `src/` directory, mirroring the structure of a standard React Native project.
If the `src/` directory doesn't exist, you can create it. Inside `src/`, you can structure your components according to your app's unique requirements.
Now, it's time to create your first component and integrate it into your Expo app.
Creating Your First Component
With your Expo project set up and the project structure understood, let's take the exciting step of creating a simple component. We'll start with a basic "Hello World" component, providing you with a solid foundation for your React Native development journey.
Step 1: Birth of a New Component
1. Begin in your project's root directory and navigate to the `src/` directory. If this directory doesn't exist, you can create it.
2. Within the `src/` directory, create a new JavaScript file and name it `HelloWorldComponent.js`.
3. Open `HelloWorldComponent.js` in your code editor and infuse it with the following code:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const HelloWorldComponent = () => {
return (
<View style={styles.container}>
<Text>Hello, World!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default HelloWorldComponent;
In this component, you import the necessary elements from React and React Native. You then define a functional component named HelloWorldComponent
that returns a simple "Hello, World!" message enclosed in a View
component. The accompanying styles ensure the message is centered on the screen.
Step 2: Integration of the Component
Now that you've crafted the `HelloWorld` component, let's integrate it into your app. Open the `App.js` file located in your project's root directory and adjust it as follows:
import React from 'react';
import { View, StyleSheet } from 'react-native';
// Import the custom component
import HelloWorldComponent from './src/HelloWorldComponent';
const App = () => {
return (
<View style={styles.container}>
<HelloWorldComponent />
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'gray',
},
});
export default App;
In this code, you import the HelloWorld
component and include it within the App
component. As a result, the "Hello, World!" message will be displayed in your app.
You can visit https://snack.expo.dev/ to explore Expo online and test it out.
Step 3: Running Your Expo App
The time has come to witness your app in action. Ensure you are inside your project's root directory within your terminal, and execute the following command:
expo start
This command initiates the Expo development server and opens a new browser window, presenting a QR code.
Step 4: Testing Your App
To test your app, you can utilize the Expo Go app on your physical device. Install Expo Go from your device's app store, launch it, and scan the QR code exhibited in the browser window.
You should now behold your app running on your device, showcasing "My First Expo App" alongside the "Hello, World!" message.
Congratulations! You've proficiently forged and tested your maiden React Native app using Expo.
Expo v/s Standard Approach: Which Is Right for You?
As you embark on your React Native development journey, it's crucial to ponder whether Expo or the standard approach is the right fit for your project. Here are a few factors to consider:
Choose Expo If:
- You are a newcomer to React Native and mobile app development.
- You prefer a streamlined development process.
- Over-the-air updates are a critical requirement for your app.
- You'd rather concentrate on building app features than configuring native modules.
Choose the Standard Approach If:
- You necessitate full control over your project's configuration.
- Specific native modules not supported by Expo are essential for your project.
- You already possess experience with React Native and desire a more customized setup.
Ultimately, both Expo and the standard approach possess their respective strengths. The ideal choice hinges on your project's prerequisites and your experience as a developer.
In our next blog post, "Understanding React Native Components and UI Elements", we'll delve deeper into the world of React Native, exploring the building blocks of your app's user interface. You'll gain a comprehensive understanding of components and UI elements, setting the stage for you to craft dynamic and interactive mobile applications.
Stay tuned for the next blog, and Happy Coding!