MailHog - Web and API based SMTP testing
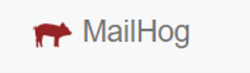
Most of the projects will have a requirement of sending and receiving mails. We have mentioned about GreenMail - Email Test Framework, in our previous article about API based SMTP testing. In this article, we discuss about MailHog - Web and API based SMTP testing. You send out a mail from your code and you can check it via web visually and also via API. Those who do API testing can check via API. Developers may want to visually verify the format of the mail. MailHog is a best bet for SMTP testing.
Git Link: https://github.com/mailhog/MailHog
License: MIT
Language: Go
MailHog is inspired by MailCatcher - A test SMTP server written in Ruby. Its feature include
- ESMTP Server with support for SMTP Auth
- Web interface to view messages (plain text, HTML or source)
- Multipart MIME support
- Option to store in-memory of MongoDB
Download the latest release
https://github.com/mailhog/MailHog/blob/master/docs/RELEASES.md
Run the below command to start the server in port 25
CMD > Mailhog -smtp-bind-addr 0.0.0.0:25
Below code is in Java to send out a mail with inline images
public static void sendMail() {
Properties props = new Properties();
props.put("mail.transport.protocol", "smtp");
props.put("mail.smtp.host", "127.0.0.1");
props.put("mail.smtp.port", "25");
String htmlContent = "<html> <head> <title></title> </head> <body> "
+ "<table align=\"left\"> <tbody> <tr> <td>"
+ " <a href=\"https://www.findbestopensource.com/images/findbestopensource-logo.jpg\""
+ " target=\"_blank\""
+ " rel=\"noopener\"><img src=\"https://www.findbestopensource.com/images/findbestopensource-logo.jpg\" width=\"300\""
+ " height=\"auto\" style=\"display:block\"/></a></td> </tr> <tr><H1> Best open source weekly newsletter. </H1></tr> </tbody> </table></body></html>";
Session mailSession = Session.getInstance(props, null);
try {
MimeMessage msg = new MimeMessage(mailSession);
msg.setFrom("abcd@example.com");
msg.setRecipients(Message.RecipientType.TO, "editor@findbestopensource.com");
msg.setSubject("Best Open Source Newsletter 14");
msg.setSentDate(new Date());
MimeMultipart content = new MimeMultipart();
BodyPart bodyPart = new MimeBodyPart();
bodyPart.setContent(htmlContent, "text/html; charset=UTF-8");
//bodyPart.setHeader("Content-Transfer-Encoding", "quoted-printable");
BodyPart bodyPart1 = new MimeBodyPart();
bodyPart1.setContent("This is a test news letter", "text/plain; charset=ISO-8859-1");
content.addBodyPart(bodyPart);
content.addBodyPart(bodyPart1);
msg.setContent(content);
Transport.send(msg);
}
catch (MessagingException e) {
e.printStackTrace();
}
}
You can check visually the message. Launch the browser and access the URL
Using API you can receive the messages in JSON format.
http://localhost:8025/api/v2/messages
MailHog is a great tool for SMTP related testing. We don't need SMTP server or mail client to test the feature. We can manually view the messages and we can also retrieve the messages via API.
Reference:
https://github.com/mailhog/MailHog